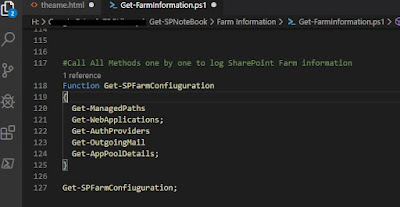
As part of upgrade process I had to document all my UAT, Prod and DR farm details.
Instead of going each server/farm and writing down all Farm Information, was able to come up with below functions with little help of Microsoft Documents about PowerShell.
In below Example I have written multiple PowerShell Functions to read each section Managed Paths , Web App Information, Out going Email information, Application Pool Details (both Web Application and Service Applications)
Each function will create Separate Out put CSV file
Application Pool Information
Below Get-AppPoolDetails Function will read and log both Web Application and Service Application Related Application Pool information in to a CSV File
Function Get-AppPoolDetails
{
$spwebApplications = Get-SPWebApplication;
#Get Web Application Related Application Pool Information
Add-Content -Path $AppPoolOutput -Value " App Pool Type|WebApplication or Service| App Pool Name| App Pool Identity"
foreach ($webApp in $spwebApplications)
{
Add-Content -Path $AppPoolOutput -Value "Web App | $($webApp.Url)| $($webApp.ApplicationPool.DisplayName)|$($webApp.ApplicationPool.ManagedAccount.Username)"
}
#Get Service Application Related Application Pool Information
$AllserviceAppApps = Get-SPServiceApplication;
foreach ($serviceApp in $AllserviceAppApps)
{
Add-Content -Path $AppPoolOutput -Value "Service App | $($serviceApp.Name)| $($serviceApp.ApplicationPool.DisplayName)|$($serviceApp.ApplicationPool.ProcessAccount.Name)"
}
}
Out Going email Details
This will read SharePoint Central Administration Web App Pool Properties about Out Going Email
Function Get-OutgoingMail
{
#Get Central Administration Web Application in to the Variable
$OutGoing = get-spwebapplication -IncludeCentralAdministration | where {$_.isAdministrationWebApplication}
Add-Content -Path $OutBoundMailOutput -Value "Out Bound Server|OutboundMailSenderAddress|OutboundMailReplyToAddress"
Add-Content -Path $OutBoundMailOutput -Value "$($OutGoing.outboundmailserviceinstance.Server.Address)|$($OutGoing.OutboundMailSenderAddress)|$($OutGoing.OutboundMailReplyToAddress)"
$outGoing="";
}
Authentication Provider Information for each Web Application
Function Get-AuthProviders
{
$spwebApplications = Get-SPWebApplication;
Add-Content -Path $WebAppAuthProviderOutput -value "WebApp Name | Web App URL |Auth Provider-DisplayName|Auth Provider-ClaimProviderName|Auth Provier-AllowAnonymous|Auth Provier-UseWindowsIntegratedAuthentication|Auth Provider-DisableKerberos"; #Error file header
foreach($webApp in $spwebApplications)
{
$authProvider = Get-SPAuthenticationProvider -WebApplication $webApp -Zone Default;
Add-Content -Path $WebAppAuthProviderOutput -value "$($webApp.Name) | $($webApp.Url) |$($authProvider.DisplayName)|$($authProvider.ClaimProviderName)|$($authProvider.AllowAnonymous)|$($authProvider.UseWindowsIntegratedAuthentication)|$($authProvider.DisableKerberos)";
}
$spwebApplications="";
}
Web Application Information and Alternative URL information for Each Web Application.
Function Get-WebApplications
{
$spwebApplications = Get-SPWebApplication;
Add-Content -Path $WebAppOutput -value "WebApp Name | Web App URL |Application Pool| AlterNate URL-Incoming URL| AlterNate URL- zone| AlterNate URL-PublicURL"; #Error file header
foreach ($webApp in $spwebApplications)
{
Write-Host $webApp;
$alternateURLs=$webApp.AlternateUrls;
foreach ($altURL in $alternateURLs)
{
Add-Content -Path $WebAppOutput -value "$($webApp.Name) | $($webApp.Url) | $($webApp.ApplicationPool.DisplayName) |$($altURL.IncomingURL)|$($altURL.Zone)|$($altURL.PublicUrl)"; #Error file
}
}
$spwebApplications="";
}
Managed Path Information for Each Web Application.
function Get-ManagedPaths
{
$SPWebApplications = Get-SPWebApplication
Add-Content -Path $managedPathOutput -value "WebApp Name | Web App URL | Managed Path |Type"; #Error file header
foreach ($webApp in $SPWebApplications)
{
$allManagedPaths = Get-SPManagedPath -WebApplication $webApp;
#Get Each Managed path for each web application.
foreach($mgdPath in $allManagedPaths)
{
Write-Host $mgdPath.Name "-" $webApp.DisplayName;
Add-Content -Path $managedPathOutput -value "$($webApp.DisplayName) | $($webApp.Url) | $($mgdPath.Name) |$($mgdPath.Type)"; #Error file header
}
}
}
Full Script with Login Function information.
Add-PSSnapin Microsoft.SharePoint.PowerShell
[System.Reflection.Assembly]::LoadWithPartialName("Microsoft.SharePoint") > $null
#Preparing Execution ID Based on Start Date and Time
cls;
$timeStamp = Get-Date;
$ExecutionIDTitle =$timeStamp.ToString("yyyy-MM-dd-HH-mm");
$ExecutionID =$timeStamp.ToString("yyyyMMddHHmm");
$outputPath = "E:\Scripts\FarmConfiguration\OutPut\$ExecutionID";
$managedPathOutput = $outputPath+"-ManagedPaths.csv";
$WebAppOutput = $outputPath+"-WebApplications-AltURLs.csv";
$WebAppAuthProviderOutput = $outputPath+"-WebApplications-AuthProviders.csv";
$OutBoundMailOutput = $outputPath+"-OutBoundMailDetails.csv";
$AppPoolOutput = $outputPath+"-appPoolDetails.csv";
Function Get-AppPoolDetails
{
$spwebApplications = Get-SPWebApplication;
#Get Web Application Related Application Pool Information
Add-Content -Path $AppPoolOutput -Value " App Pool Type|WebApplication or Service| App Pool Name| App Pool Identity"
foreach ($webApp in $spwebApplications)
{
Add-Content -Path $AppPoolOutput -Value "Web App | $($webApp.Url)| $($webApp.ApplicationPool.DisplayName)|$($webApp.ApplicationPool.ManagedAccount.Username)"
}
#Get Service Application Related Application Pool Information
$AllserviceAppApps = Get-SPServiceApplication;
foreach ($serviceApp in $AllserviceAppApps)
{
Add-Content -Path $AppPoolOutput -Value "Service App | $($serviceApp.Name)| $($serviceApp.ApplicationPool.DisplayName)|$($serviceApp.ApplicationPool.ProcessAccount.Name)"
}
}
Function Get-OutgoingMail
{
#Get Central Administration Web Application in to the Variable
$OutGoing = get-spwebapplication -IncludeCentralAdministration | where {$_.isAdministrationWebApplication}
Add-Content -Path $OutBoundMailOutput -Value "Out Bound Server|OutboundMailSenderAddress|OutboundMailReplyToAddress"
Add-Content -Path $OutBoundMailOutput -Value "$($OutGoing.outboundmailserviceinstance.Server.Address)|$($OutGoing.OutboundMailSenderAddress)|$($OutGoing.OutboundMailReplyToAddress)"
$outGoing="";
}
Function Get-AuthProviders
{
$spwebApplications = Get-SPWebApplication;
Add-Content -Path $WebAppAuthProviderOutput -value "WebApp Name | Web App URL |Auth Provider-DisplayName|Auth Provider-ClaimProviderName|Auth Provier-AllowAnonymous|Auth Provier-UseWindowsIntegratedAuthentication|Auth Provider-DisableKerberos"; #Error file header
foreach($webApp in $spwebApplications)
{
$authProvider = Get-SPAuthenticationProvider -WebApplication $webApp -Zone Default;
Add-Content -Path $WebAppAuthProviderOutput -value "$($webApp.Name) | $($webApp.Url) |$($authProvider.DisplayName)|$($authProvider.ClaimProviderName)|$($authProvider.AllowAnonymous)|$($authProvider.UseWindowsIntegratedAuthentication)|$($authProvider.DisableKerberos)"; #Error file header
}
$spwebApplications="";
}
Function Get-WebApplications
{
$spwebApplications = Get-SPWebApplication;
Add-Content -Path $WebAppOutput -value "WebApp Name | Web App URL |Application Pool| AlterNate URL-Incoming URL| AlterNate URL- zone| AlterNate URL-PublicURL"; #Error file header
foreach ($webApp in $spwebApplications)
{
Write-Host $webApp;
$alternateURLs=$webApp.AlternateUrls;
foreach ($altURL in $alternateURLs)
{
Add-Content -Path $WebAppOutput -value "$($webApp.Name) | $($webApp.Url) | $($webApp.ApplicationPool.DisplayName) |$($altURL.IncomingURL)|$($altURL.Zone)|$($altURL.PublicUrl)"; #Error file
}
}
$spwebApplications="";
}
function Get-ManagedPaths
{
$SPWebApplications = Get-SPWebApplication
Add-Content -Path $managedPathOutput -value "WebApp Name | Web App URL | Managed Path |Type"; #Error file header
foreach ($webApp in $SPWebApplications)
{
$allManagedPaths = Get-SPManagedPath -WebApplication $webApp;
#Get Each Managed path for each web application.
foreach($mgdPath in $allManagedPaths)
{
Write-Host $mgdPath.Name "-" $webApp.DisplayName;
Add-Content -Path $managedPathOutput -value "$($webApp.DisplayName) | $($webApp.Url) | $($mgdPath.Name) |$($mgdPath.Type)"; #Error file header
}
}
}
#Call All Methods one by one to log SharePoint Farm information
Get-ManagedPaths
Get-WebApplications;
Get-AuthProviders
Get-OutgoingMail
Get-AppPoolDetails;
Once Executed the entire Script, you will get your output files in Output Folder as follows
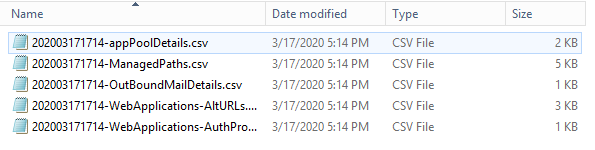